20190311
this关键字
20190313
static关键字
代码块
public class Demo03
{
{
System.out.println("构造块");
}
public Demo03()
{
System.out.println("构造函数");
}
static
{
System.out.println("静态代码块");
}
public static void main(String[] args)
{
System.out.println("普通块");
}
}
public class Demo04
{
public static void main(String[] args)
{
new Demo();
new Demo();
new Demo();
}
}
/*结果:
静态代码块
构造块
构造函数
构造块
构造函数
构造块
构造函数
运行结果:
1.静态块优先于主方法执行。
2.静态块只执行一次。
3.构造快先于构造方法执行。
*/
构造方法私有化
class Singleton
{
static Singleton instance=new Singleton();
private Singleton(){}
public void print()
{
System.out.println("hello, world!");
}
}
public class Demo06
{
public static void main(String[] args)
{
Singleton s=null;
s=Singleton.instance;
s.print();
}
}
/*构造方法私有化以后,
不能在外部使用new在实例化对象了,
但是可以使用static获取类的实例。
*/
对象数组
Person[] p=new Person[3];
内部类:在一个类的内部定义的类
class Outer{
private String info="hello, world";
class Inner
{
public void print()
{
System.out.println(info);
}
}
public void fun()
{
new Inner().print();
}
}
public class Demo08{
public static void main(String[] args){
new Outer().fun();
}
}
20190320
继承的基本概念//java只能单继承
class 派生类名 extends 基类 {}
public class Student extends Person{}
方法的覆写:在继承的关系中,所谓方法覆写及时指子类定义了
与父类同名的方法,但是在方法覆写时必须考虑到权限,即被子
类覆写的方法不能拥有比父类更加严格的访问权限。
在Java中,访问权限从小到大: private, default, protected, public.
如果父类的方法时public的,则子类必须时public
super关键字
序号 区别 this super
1 属性 访问本类的属性,如果本类中没有此属性,则从父类中查找 访问父类中的属性
2 方法 访问本类的方法,如果本类中没有此方法,则从父类中查找 直接访问父类中的方法
3 构造函数 调用本类的构造函数,必须放在构造方法的首行 调用父类的构造方法,必须放在子类构造方法的首行
4 特殊 表示当前对象 无此概念
final关键字
final的表示最终,final可以声明类、属性、方法、注意以下几点:
使用final声明类,则类不能有子类
使用final声明方法,则不能被子类覆写
声明final声明变量,则是常量,不可修改。
抽象类
只声明,不实现的方法,用关键字abstract声明
abstract和final不能同时存在!
抽象类允许构造函数。
如果类中有abstract方法,则类一定用abstract修饰,但是类中
没有abstract方法,类也可以使用abstract修饰,使之成为抽象类。
20190320
接口
interface A
{
public static final String name="ycwang";
public void print();
public String getInfo();
}
class B implements A
{
public void print(){
System.out.println("hello, world!!!");
}
public String getInfo(){
return "hello "+name+"!";
}
}
public class Demo01
{
public static void main(String[] args)
{
B b= new B();
b.print();
System.out.println(b.getInfo());
}
}
只能继承一个,可以接口多个
class C implements A,B
{...}
对象多态性
向上转型:子类对象 -> 父类对象 (自动) //优点:不用每次去重写子类中与父类重名的方法了https://blog.csdn.net/qq_31655965/article/details/54746235
class A{
public void fun1(){
System.out.println("1、A类中的fun1()");
}
public void fun2(){
this.fun1();
}
}
class B extends A{
public void fun1(){
System.out.println("2、B类中的fun1()");
}
public void fun3(){
System.out.println("3、B类中的fun3()");
}
}
public class Demo01 {
public static void main(String[] args) {
A a=new B();
a.fun2();//执行的永远是被覆写过的子类的方法。
}
}
向下转型:父类对象 -> 子类对象 (强制)
抽象类的应用:
abstract class Person
{
private String name;
private int age;
public String getName()
{
return name;
}
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public int getAge() {
return age;
}
public void say(){
System.out.println(this.getContent());
}
public abstract String getContent();
}
public class Demo01
{
public static void main(String[] args) {
}
}
20190327
抽象类与接口的应用
要求:设计两个类,学生和工人。对这些类发送同样的消息,根据接受对象的不同
,执行不同的操作。
abstract class Person{
private String name;
private int age;
public Person(String name,int age) {
this.name=name;
this.age=age;
}
public String getName() {
return this.name;
}
public int getAge() {
return this.age;
}
public void say() {
System.out.println(this.getContent());
}
public abstract String getContent();
}
class Student extends Person{
private float score;
public Student(String name,int age,float score){
super(name,age);
this.score=score;
}
public String getContent() {
return "学生信息==> 姓名:"+super.getName()+
",年龄:"+super.getAge()+
",成绩:"+this.score;
}
}
class Worker extends Person{
private float salary;
public Worker(String name,int age,float salary) {
super(name,age);
this.salary=salary;
}
public String getContent() {
return "工人信息==> 姓名:"+super.getName()+
",年龄:"+super.getAge()+
",工资:"+this.salary;
}
}
public class Demo01 {
public static void main(String[] args) {
Person p1=new Student("张三",20,90.0f);
Person p2=new Worker("李四",30,3000.0f);
p1.say();
p2.say();
}
}
接口的实际应用---制定标准
interface USB{
public void start();
public void stop();
}
class Flash implements USB{
public void start() {
System.out.println("插入U盘!");
}
public void stop() {
System.out.println("拔出U盘!");
}
}
class Printer implements USB{
public void start() {
System.out.println("开始打印!");
}
public void stop() {
System.out.println("打印完成!");
}
}
class Mp3 implements USB{
public void start() {
System.out.println("开始播放!");
}
public void stop() {
System.out.println("停止播放!");
}
}
class Computer{
public void plugin(USB usb) {
usb.start();
System.out.println("USB设备正在工作。。。。");
usb.stop();
}
}
public class Demo02 {
public static void main(String[] args) {
Computer c=new Computer();
Flash f=new Flash();
Mp3 m=new Mp3();
Printer p=new Printer();
c.plugin(f);
c.plugin(m);
c.plugin(p);
}
}
设计模式--工厂模式
interface Fruit{
public void eat();
}
class Apple implements Fruit{
public void eat() {
System.out.println("吃苹果!");
}
}
class Orange implements Fruit{
public void eat() {
System.out.println("吃橘子!");
}
}
class Factory{
public static Fruit getInstance(String classname){
Fruit f=null;
if("Apple".equals(classname)) {
f=new Apple();
}
if("Orange".equals(classname)) {
f=new Orange();
}
return f;
}
}
public class Demo03 {
public static void main(String[] args) {
Fruit f=null;
f=Factory.getInstance(args[0]);
f.eat();
}
}
设计模式-代理模式
interface Network{
public void browse();
}
class Real implements Network{
public void browse() {
System.out.println("上网浏览信息!");
}
}
class Proxy implements Network{
private Network network;
public Proxy(Network network)
{
this.network=network;
}
public void check() {
System.out.println("检查用户是否合法!");
}
public void browse() {
this.check();
this.network.browse();
System.out.println("上网结束");
}
}
public class Demo04 {
public static void main(String[] args) {
Network net=null;
net=new Proxy(new Real());
net.browse();
}
}
票贩子替买火车票
interface Ticket{
public void buyTicket();
}
class Real implements Ticket{
public void buyTicket() {
System.out.println("买到火车票!");
}
}
class Proxy implements Ticket{
private Ticket ticket;
public Proxy(Ticket ticket) {
this.ticket=ticket;
}
public void check() {
System.out.println("拿到客户的身份证信息!");
}
public void send() {
System.out.println("把票送给客户!");
}
public void buyTicket() {
this.check();
this.ticket.buyTicket();
this.send();
}
}
public class ProxyDemo {
public static void main(String[] args) {
Ticket ticket=null;
ticket=new Proxy(new Real());
ticket.buyTicket();
}
}
设计模式---适配器模式
interface Window{
public void open();
public void max();
public void min();
public void close();
}
abstract class WindowAdapter implements Window{
public void open() {}
public void max() {}
public void min() {}
public void close() {}
}
class WinApp extends WindowAdapter{
public void open() {
System.out.println("打开窗口");
}
}
public class AdapterDemo {
public static void main(String[] args) {
WinApp w=new WinApp();
w.open();
}
}
Object类
Java中所有类的公共父类Object。
1.toString()方法:
比如打印对象的toString()返回的字符串,显示对象信息:
class Person {
private String name;
private int age;
public Person(String name, int age) {
super();
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String toString() {
return "人员信息:\n\t|姓名:"+ this.name+"\n\t"+
"|年龄:"+this.age;
}
}
public class Demo01 {
public static void main(String[] args) {
Person p=new Person("张三",30);
System.out.println(p);
}
}
2.equals()方法:
class Person {
private String name;
private int age;
public Person(String name, int age) {
super();
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String toString() {
return "人员信息:\n\t|姓名:"+ this.name+"\n\t"+
"|年龄:"+this.age;
}
public boolean equals(Object obj) {
if(this == obj) {
return true;
}
if(!(obj instanceof Person)) {
return false;
}
Person p=(Person)obj;
if(p.name.equals(this.name) && (p.age==this.age)){
return true;
}else {
return false;
}
}
}
public class Demo01 {
public static void main(String[] args) {
Person p1=new Person("张三",30);
Person p2=new Person("张三",30);
System.out.println(p1.equals(p2));
}
}
包装类:
序号 基本数据类型 包装类
1 int Integer
2 char Character
3 short Short
4 long Long
5 float Float
6 double Double
7 boolean Boolean
8 byte Byte
包装类和基本类型之间可以进行装箱操作和拆箱操作
int x=30;
Integer i=new Integer(x);//装箱操作
int y=i.intValue();//拆箱操作
System.out.println(y+10);
在高版本的java(1.5以后),可以自动装箱和拆箱。
int x=30;
Integer i=x;//自动装箱操作
int y=i; //自动拆箱操作
System.out.println(y+10);
20190401
异常的捕获及处理
1 基本概念
在进行异常处理执勤前,先看一下如下的程序:
int i=10;
int j=0;
int tmp=i/j;
System.out.println("两个数相除结果:"+tmp);
System.out.println("***********计算结束*************");
因为除0,程序没有正常结束。
Exception in thread "main" java.lang.ArithmeticException: / by zero
在java中,使用 try{ 。。。。。 }catch{ 。。。。。。。 },比如可以捕获算数异常:
int i=10;
int j=0;
System.out.println("***********计算开始*************");
try{
int tmp=i/j;
System.out.println("两个数相除结果:"+tmp);
}catch(ArithmeticException e){
System.out.println("算数异常,除0");
}
System.out.println("***********计算结束*************");
如果有多个异常,可以多次捕获:
System.out.println("***********计算开始*************");
try{
String x=args[0];
String y=args[1];
int i=Integer.parseInt(x);
int j=Integer.parseInt(y);
int tmp=i/j;
System.out.println("两个数相除结果:"+tmp);
}catch(ArithmeticException e){
System.out.println("算数异常,除0");
}catch(ArrayIndexOutOfBoundsException e){
System.out.println("发生了数组越界异常,参数个数不对!");
}catch(NumberFormatException e){
System.out.println("输入的参数不是数字。");
}
System.out.println("***********计算结束*************");
异常类的继承结构:
1.Throwable
2. Exception和Error
Java异常处理机制
catch(Exception e){
System.out.println("发生了异常。"+e.getMessage());
}catch(ArithmeticException e){
System.out.println("算数异常,除0");
}catch(ArrayIndexOutOfBoundsException e){
System.out.println("发生了数组越界异常,参数个数不对!");
}catch(NumberFormatException e){
System.out.println("输入的参数不是数字。");
}
2 throws关键字
class Math{
public int div(int i, int j) throws Exception {
int tmp=i/j;
return tmp;
}
}
public class Demo5 {
public static void main(String[] args) {
Math m=new Math();
try {
int t=m.div(10, 0);
} catch (Exception e) {
e.printStackTrace();
}
}
}
3 throw关键字
使用try{ }catch{}finally{} 和 throws、throw给出标准化的异常处理框架
class Maths{
public int div(int i,int j) throws Exception {
System.out.println("======计算开始======");
int tmp=0;
try{
tmp=i/j;
}catch(Exception e) {
throw e;
}finally {
System.out.println("======计算结束======");
}
return tmp;
}
}
public class Demo7 {
public static void main(String[] args) {
Maths m=new Maths();
try {
System.out.println("除法操作:"+m.div(10, 0));
}catch(Exception e) {
System.out.println("异常产生:"+e);
}
}
}
4 自定义异常
class MyException extends Exception{
public MyException(String msg)
{
super(msg);
}
}
public class Demo2 {
public static void main(String[] args)
{
try {
throw new MyException("自定义异常!");
} catch (MyException e) {
System.out.println(e);;
}
}
}
20190404
1 包的概念
就是个文件夹
包的定义:
package 包名称.子包名称;
比如如下一个类:
package cn.tjut;
public class Demo01
{
public static void main(String[] args){
System.out.println("hello");
}
}
编译方法:
javac -d . Demo01.java
运行方法:
java cn.tjut.Demo01
.表示当前目录,..表示当前目录的上级目录
例子:
package cn.tjut.a;
import cn.tjut.b.Person;
public class Demo02
{
public static void main(String[] args){
Person p=new Person("张三",30);
System.out.println(p);
}
}
被调用的类在另一个包中:
package cn.tjut.b;
public class Person
{
private String name;
private int age;
public Person(String name,int age){
this.name=name;
this.age=age;
}
public String toString(){
return "姓名:"+this.name+", 年龄:"+this.age;
}
}
注意编译顺序,要先编译被调用的类,再编译调用者的类。
public 权限表示跨文件夹也可以访问。
如果要导入的类很多,而且在同一个文件夹下,则可以使用通配符*来统一导入。
package cn.tjut.a;
import cn.tjut.b.*;
public class Demo02
{
public static void main(String[] args){
Person p=new Person("张三",30);
System.out.println(p);
}
}
2 系统常见包
序号 包名称 作用
1 java.lang 最主要的包,是缺省的包,比如String类
2 java.lang.reflect 反射机制包
3 java.util 工具包
4 java.text 文本处理,国际化
5 java.sql 数据库操作
6 java.net 网络编程
7 java.io 输出输入包
8 java.awt 抽象窗口工具集,编写窗口应用程序
9 javax.swing 建立用户图形界面GUI编程
3 静态导入
被调用的类中的方法是static静态的。
调用者: import static 包.子包.类.*;
package cn.tjut.c;
public class Operate
{
public static int add(int i,int j){
return i+j;
}
public static int sub(int i,int j){
return i-j;
}
public static int mul(int i,int j){
return i*j;
}
public static int div(int i,int j){
return i/j;
}
}
package cn.tjut.d;
import static cn.tjut.c.Operate.*;
public class Demo03
{
public static void main(String[] args){
System.out.println("3+3="+add(3,3));
System.out.println("3-2="+sub(3,2));
System.out.println("3*3="+mul(3,3));
System.out.println("3/3="+div(3,3));
}
}
4 访问权限控制
访问权限: private, default , protected, public 4种。
1 private:私有访问,只能在本类中访问。类外部不能访问。
2 default:如果没有写访问权限控制符,则是default
3 proteced: 受保护的访问权限。
4 public:公共访问权限.
范围 private default protected public
类内部 √ √ √ √
一个包中 √ √ √
不同包的子类 √ √
不同包的非子类 √
20190408
访问权限控制
package cn.tjut.a;
import cn.tjut.b.*;
public class Demo01 {
public static void main(String[] args) {
Test t=new Test();// 在类内部。
//t.fun();
//System.out.println(t.pri);//private变量不可见
// System.out.println(t.def);
// System.out.println(t.pro);
// System.out.println(t.pub); //验证了第二行
Testb tb=new Testb();
//tb.fun(); //验证了第三行
Testc tc=new Testc();
tc.fun(); //验证了第四行
}
}
package cn.tjut.a;
public class Test {
private String pri="private variable";
String def="default variable";
protected String pro="protected variable";
public String pub="public variable";
public void fun() {
System.out.println(pri);
System.out.println(def);
System.out.println(pro);
System.out.println(pub);
}
}
package cn.tjut.b;
import cn.tjut.a.*;
public class Testb extends Test {
public void fun() {
// System.out.println(pri);
// System.out.println(def);
System.out.println(pro);
System.out.println(pub);
}
}
package cn.tjut.b;
import cn.tjut.a.*;
public class Testc {
public void fun() {
Test t=new Test();
// System.out.println(t.pri);
// System.out.println(t.def);
// System.out.println(t.pro);
System.out.println(t.pub);
}
}
多线程
Java实现多线程代码有两种手段:
继承Thread类
实现Runnable接口
先考察如下程序:
package cn.tjut;
class MyThread extends Thread{
private String name;
public MyThread(String name) {
this.name=name;
}
public void run() {
for(int i=0;i<100;i++) {
System.out.println(name+"运行:i="+i);
}
}
}
public class Demo01 {
public static void main(String[] args) {
MyThread mt1=new MyThread("线程A");
MyThread mt2=new MyThread("线程B");
mt1.run();
mt2.run();
}
}
run换为tart:
package cn.tjut;
class MyThread extends Thread{
private String name;
public MyThread(String name) {
this.name=name;
}
public void run() {
for(int i=0;i<100;i++) {
System.out.println(name+"运行:i="+i);
}
}
}
public class Demo01 {
public static void main(String[] args) {
MyThread mt1=new MyThread("线程A");
MyThread mt2=new MyThread("线程B");
mt1.start();
mt2.start();
}
}
Runnable实现多线程:
package cn.tjut.demo3;
class MyThread implements Runnable{
private String name;
public MyThread(String name) {
this.name=name;
}
public void run() {
for (int i=0;i<=100;i++) {
System.out.println(name+"运行:i="+i);
}
}
}
public class Demo3 {
public static void main(String[] args) {
MyThread mt1=new MyThread("线程A");
MyThread mt2=new MyThread("线程B");
Thread t1=new Thread(mt1);
Thread t2=new Thread(mt2);
t1.start();
t2.start();
}
}
Thread类和Runnable接口
使用Thread类,不能共享变量资源,而Runnable方式可以共享。
用两种方式实现的卖票程序如下:
package cn.tjut.demo4;
public class MyThread extends Thread {
private int ticket=5;
public void run() {
for(int i=0;i<=100;i++) {
if(ticket>=0) {
System.out.println("卖票:ticket="+ticket--);
}
}
}
}
package cn.tjut.demo4;
public class Demo04 {
public static void main(String[] args) {
MyThread mt1=new MyThread();
MyThread mt2=new MyThread();
MyThread mt3=new MyThread();
mt1.start();
mt2.start();
mt3.start();
}
}
20190411
多线程实现的两种方法
继承Thread类
实现Runnable接口
取得和设置线程名称:
package cn.tjut.namedemo;
public class ThreadNameDemo01 {
public static void main(String[] args) {
MyThread mt=new MyThread();
Thread t1=new Thread(mt,"线程A");
Thread t2=new Thread(mt,"线程B");
Thread t3=new Thread(mt,"线程C");
t1.start();
t2.start();
t3.start();
}
}
package cn.tjut.namedemo;
public class MyThread implements Runnable{
public void run(){
for(int i=0;i<=10;i++){
System.out.println(Thread.currentThread().getName()+"线程在运行");
}
}
}
对于Java程序,每次运行系统都启动一个JVM进程。至少启动两个线程:main, gc
线程的休眠:
package cn.tjut.sleepdemo;
public class ThreadSleepDemo01 {
public static void main(String[] args) {
MyThread mt=new MyThread();
new Thread(mt,"线程A").start();
new Thread(mt,"线程B").start();
}
}
package cn.tjut.sleepdemo;
public class MyThread implements Runnable{
public void run(){
for(int i=0;i<=10;i++){
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName()+"线程在运行");
}
}
}
线程的中断:
package cn.tjut.interruptdemo;
public class InterruptDemo {
public static void main(String[] args) {
MyThread mt=new MyThread();
Thread thread=new Thread(mt,"线程A");
thread.start();
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
thread.interrupt();
}
}
package cn.tjut.interruptdemo;
public class MyThread implements Runnable{
public void run(){
System.out.println("1.进入run()方法");
try {
System.out.println("2.线程休眠20秒");
Thread.sleep(20000);
System.out.println("3.线程休眠正常结束");
} catch (InterruptedException e) {
System.out.println("4.线程休眠被中断");
}
System.out.println("5.正常结束run()方法");
}
}
线程的优先级:
package cn.tjut.prioritydemo;
public class PriorityDemo01 {
public static void main(String[] args) {
System.out.println("main的优先级:"+Thread.currentThread().getPriority());
System.out.println("MAX_PRIORITY:"+Thread.MAX_PRIORITY);
System.out.println("MIN_PRIORITY:"+Thread.MIN_PRIORITY);
System.out.println("NORM_PRIORITY:"+Thread.NORM_PRIORITY);
}
}
20190415
线程同步
未同步时:
package cn.tjut.threaddemo01;
public class ThreadDemo01 {
public static void main(String[] args) {
MyThread mt=new MyThread();
Thread t1=new Thread(mt);
Thread t2=new Thread(mt);
Thread t3=new Thread(mt);
t1.start();
t2.start();
t3.start();
}
}
package cn.tjut.threaddemo01;
public class MyThread implements Runnable {
private int ticket=5;
public void run() {
for(int i=0;i<=100;i++) {
if(ticket>=0) {
try {
Thread.sleep(300);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("卖票:"+ticket--);
}
}
}
}
同步关键字为 synchronized
一般封装当前类,使用 synchronized(this)
package cn.tjut.threaddemo01;
public class MyThread implements Runnable {
private int ticket = 5;
public void run() {
for (int i = 0; i <= 100; i++) {
synchronized (this) {
if (ticket >= 0) {
try {
Thread.sleep(300);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("卖票:" + ticket--);
}
}
}
}
}
另一种是使用同步方法:
package cn.tjut.threaddemo01;
public class MyThread implements Runnable {
private int ticket = 5;
public void run() {
for (int i = 0; i <= 100; i++) {
this.sale();
}
}
public synchronized void sale() {
if (ticket >= 0) {
try {
Thread.sleep(300);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("卖票:" + ticket--);
}
}
}
死锁
同步使用过度,容易产生死锁。
package cn.tjut.threaddemo01;
public class ThreadDeadLock implements Runnable {
private static Zhangsan zs=new Zhangsan();
private static Lisi ls=new Lisi();
public boolean flag=false;
public void run() {
if(flag) {
synchronized(zs){
zs.say();
try {
Thread.sleep(500);
} catch (InterruptedException e) {
e.printStackTrace();
}
synchronized(ls) {zs.get();
}
}
}else{
synchronized(ls) {
ls.say();
try {
Thread.sleep(500);
} catch (InterruptedException e) {
e.printStackTrace();
}
synchronized(zs) {
ls.get();
}
}
}
}
public static void main(String[] args) {
ThreadDeadLock t1=new ThreadDeadLock();
ThreadDeadLock t2=new ThreadDeadLock();
t1.flag=true;
t2.flag=false;
Thread tA=new Thread(t1);
Thread tB=new Thread(t2);
tA.start();
tB.start();
}
}
泛型
package cn.tjut.genericsdemo02;
public class Point<T> {
private T x;
private T y;
public T getX() {
return x;
}
public void setX(T x) {
this.x = x;
}
public T getY() {
return y;
}
public void setY(T y) {
this.y = y;
}
}
调用程序如下:
package cn.tjut.genericsdemo02;
public class Demo01 {
public static void main(String[] args) {
Point<Integer> p=new Point<Integer>();
p.setX(10);
p.setY(20);
int x=(Integer)p.getX();
int y=(Integer)p.getY();
System.out.println("x坐标:"+x);
System.out.println("y坐标:"+y);
}
}
泛型应用中的构造方法
package cn.tjut.genericsdemo02;
public class Point<T> {
private T x;
private T y;
public Point(T x, T y) {
this.x = x;
this.y = y;
}
public T getX() {
return x;
}
public void setX(T x) {
this.x = x;
}
public T getY() {
return y;
}
public void setY(T y) {
this.y = y;
}
}
package cn.tjut.genericsdemo02;
public class Demo01 {
public static void main(String[] args) {
Point<Integer> p=new Point<Integer>(10,20);
int x=(Integer)p.getX();
int y=(Integer)p.getY();
System.out.println("x坐标:"+x);
System.out.println("y坐标:"+y);
}
}
指定多个泛型:
package cn.tjut.genericsdemo02;
public class Notepad<K,V> {
private K key;
private V value;
public K getKey() {
return key;
}
public void setKey(K key) {
this.key = key;
}
public V getValue() {
return value;
}
public void setValue(V value) {
this.value = value;
}
}
package cn.tjut.genericsdemo02;
public class Demo02 {
public static void main(String[] args) {
Notepad<String, Integer> n=new Notepad<String, Integer>();
n.setKey("TEST");
n.setValue(123);
System.out.println(n.getKey()+"===>"+n.getValue());
}
}
20190418
泛型的擦除
Point<Object> p=new Point<Object>();
泛型的通配符 用?表示任意类型
public static void main(String[] args) {
Point<Object> p1=new Point<Object>();
Point<Integer> p2=new Point<Integer>();
fun(p1);
fun(p2);
}
public static void fun(Point<?> po){
System.out.println(po.getX());
System.err.println(po.getY());
}
泛型的上限
指定操作泛型的最大操作父类
public class Point<T extends Number>
则以下的程序都正常运行
Point<Integer> p1=new Point<Integer>();
Point<Float> p2=new Point<Float>();
Point<Number> p3=new Point<Number>();
假设Point类本身泛型不设上限
public class Point<T>
但是调用
public static void main(String[] args) {
Point<Integer> p1=new Point<Integer>();
Point<Object> p2=new Point<Object>();
Point<Number> p3=new Point<Number>();
fun(p1);
fun(p2);
fun(p3);
}
public static void fun(Point<? extends Number> po){
System.out.println(po.getX());
System.out.println(po.getY());
}
则P2点调用出错
泛型的下限
泛型下限指的是只能设置其具体的类或者父类
public static void main(String[] args) {
Point<String> p1=new Point<String>();
Point<Object> p2=new Point<Object>();
Point<Number> p3=new Point<Number>();
fun(p1);
fun(p2);
fun(p3);
}
public static void fun(Point<? super String> po){
System.out.println(po.getX());
System.out.println(po.getY());
}
泛型接口
语法:
Interface 接口名称<泛型类型,泛型类型。。。>{ }
接口泛型的范例1:
package cn.tjut.gendemo03;
public interface Demo<T> {
public void print(T param);
}
实现类;
package cn.tjut.gendemo03;
public class DemoImpl1<T> implements Demo<T> {
public void print(T param) {
System.out.println("param="+param);
}
}
调用:
package cn.tjut.gendemo03;
public class Demo01 {
public static void main(String[] args) {
Demo<String> demo=new DemoImpl1<String>();
demo.print("hello");
}
}
接口泛型的范例2:
package cn.tjut.gendemo03;
public class DemoImpl2 implements Demo<DemoImpl2> {
public void print(DemoImpl2 param) {
System.out.println("param="+param);
}
public String toString(){
return "hello, demo2";
}
}
package cn.tjut.gendemo03;
public class DemoImpl2 implements Demo<DemoImpl2> {
public void print(DemoImpl2 param) {
System.out.println("param="+param);
}
public String toString(){
return "hello, demo2";
}
}
package cn.tjut.gendemo03;
public class Demo02 {
public static void main(String[] args) {
Demo<DemoImpl2> demo=new DemoImpl2();
demo.print(new DemoImpl2());
}
}
泛型方法:泛型写在方法上,调用是不用写了就
package cn.tjut.gendemo04;
public class Demo {
public <T> T print(T param){
return param;
}
}
package cn.tjut.gendemo04;
public class Demo01 {
public static void main(String[] args) {
Demo d=new Demo();
System.out.println(d.print("hello"));
}
}
20190422
泛型嵌套范例
public class Info<T> {
private T param;
public T getParam() {
return param;
}
public void setParam(T param) {
this.param = param;
}
}
public class Person<T> {
private T info;
public T getInfo() {
return info;
}
public void setInfo(T info) {
this.info = info;
}
}
public class Test {
public static void main(String[] args) {
Person<Info<String>> p=new Person<Info<String>>();
p.setInfo(new Info<String>());
p.getInfo().setParam("hello");
System.out.println(p.getInfo().getParam());
}
}
Java常用类库
1 String类
String s=new String("hello, world!!!");
System.out.println(s.charAt(4)); //o
System.out.println(s.concat(" TJUT")); //hello, world!!! TJUT
System.out.println(s.startsWith("h")); //true
System.out.println(s.endsWith("!")); //true
byte[] b=s.getBytes();
for(int i=1;i<b.length;i++) {
System.out.println(b[i]);
}
System.out.println(s.indexOf("o")); //4
System.out.println(s.length()); //15
String s="abcdefghijklmnopqrstuvwxyz";
System.out.println(s.substring(6)); //ghijklmnopqrstuvwxyz
System.out.println(s.toUpperCase()); //ABCDEFGHIJKLMNOPQRSTUVWXYZ
2 StringBuffer类
自己写倒置程序:
package cn.tjut;
class Demo{
public static void main(String[] args) {
StringBuffer buf=new StringBuffer();
String in = "HelloWorld";
System.out.println(in.length());
int i = 0;
while(i<=in.length()-1) {
buf.append(in.charAt(in.length()-i-1));
i++;
}
System.out.println(buf);
}
}
buf.append("hello ");
3 Runtime类
Runtime run=Runtime.getRuntime();
System.out.println("系统最大内存:"+run.maxMemory());
System.out.println("系统剩余内存:"+run.freeMemory());
20190425
4 国际化程序
Local loc = Local
5 System类
获取时间
long time=System.currentTimeMillis();
System.out.println(time);
获取当前系统属性
System.getProperties().list(System.out);
回收垃圾内存
System.gc();
20190428
日期操作类
1 DATA类
Date date=new Date();
System.out.println("当前日期:" + date);
2 Calendar类(这个类是个抽象类,只能通过其子类实例化对象)(util包)
Calendar calendar=new GregorianCalendar();
System.out.println("年: " + calendar.get(Calendar.YEAR));
System.out.println("月: " + (calendar.get(Calendar.MONTH)+1));
System.out.println("日: " + calendar.get(Calendar.DAY_OF_MONTH));
System.out.println("时: " + calendar.get(Calendar.HOUR_OF_DAY));
System.out.println("分: " + calendar.get(Calendar.MINUTE));
System.out.println("秒: " + calendar.get(Calendar.SECOND));
System.out.println("毫秒: " + calendar.get(Calendar.MILLISECOND));
3 SimpleDateFormat类
Math类(无包)
System.out.println("PI="+Math.PI);
System.out.println("e="+Math.E);
Math.random():发生0到1之间的随机数
20190429
Random类(util包)
Random r=new Random();
double x=r.nextDouble();
BigInteger类(import java.math.BigInteger;)
String s1="98765432109876543210987654321098765432109876543210";
String s2="12345678901234567890123456789012345678901234567890";
BigInteger b1=new BigInteger(s1);
BigInteger b2=new BigInteger(s2);
System.out.println("b1+b2="+b1.add(b2));
System.out.println("b1-b2="+b1.subtract(b2));
System.out.println("b1*b2="+b1.multiply(b2));
BigInteger[] r=b1.divideAndRemainder(b2);
System.out.println("b1/b2的商="+r[0]);
System.out.println("b1/b2的余数="+r[1]);
20190506
Java IO(import java.io.*;)
字节流和字符流,Writer Reader
1 InputStream
package cn.tjut;
import java.io.*;
class Demo{
public static void main(String[] args) throws Exception {
File file = new File("d:"+File.separator+"demo.txt");
file.createNewFile();
InputStream is = new FileInputStream(file);
byte[] s = new byte[1024];
is.read(s);
is.close();
System.out.println(new String(s));
}
}
2 OutputStream
package cn.tjut;
import java.io.*;
class Demo{
public static void main(String[] args) throws Exception {
File file = new File("d:"+File.separator+"demo.txt");
if(file.exists()) {
file.delete();
}
file.createNewFile();
OutputStream os = new FileOutputStream(file);
os.write("12314214235215315".getBytes());
os.close();
}
}
3 Reader
package cn.tjut;
import java.io.*;
class Demo{
public static void main(String[] args) throws Exception {
File file = new File("d:"+File.separator+"demo.txt");
Reader is = new FileReader(file);
char[] s = new char[1024];
is.read(s);
System.out.println(s);
is.close();
}
}
4 Writer
package cn.tjut;
import java.io.*;
class Demo{
public static void main(String[] args) throws Exception {
File file = new File("d:"+File.separator+"demo.txt");
if(file.exists()) {
file.delete();
}
file.createNewFile();
Writer os = new FileWriter(file);
os.write("test");
os.close();
}
}
5 file其它操作
File file=new File("e:"+ File.separator + "demo.txt")
File file2=new File("e:"+ File.separator + "aaa")
file.createNewFile()
file.delete()
file.exists()
file1.isFile()
file2.isDirectory()
String[] f=file.list()//列出文件名和文件夹
File[] f=file.listFiles();//列出包含文件路径的文件名和文件夹
RandomAccessFile类
File file=new File("d:"+ File.separator + "demo.txt")
RandomAccessFile raf = new RandomAccessFile(file,"rw");
raf.writeBytes("123");
raf.writeInt(123);
File file=new File("d:"+ File.separator + "demo.txt");
RandomAccessFile raf = new RandomAccessFile(file,"r");
raf.skipBytes(3);
String b = raf.readLine();
System.out.println(b);
raf.close();
20190509
JavaIO
20190516
ByteArrayInputStream ByteArrayOutputStream内存操作流
ByteArrayInputStream bis = new ByteArrayInputStream("hello".getBytes());
ByteArrayOutputStream bos = new ByteArrayOutputStream();
int temp=0;
while((temp=bis.read()) !=-1) {
char c=(char)temp;
bos.write(Character.toUpperCase(c));
}
String newStr=bos.toString();
System.out.println(newStr);
管道流
PipedInputStream PipedOutputStream
打印流 PrintStream
Scanner
Scanner scan = new Scanner(System.in);
if (scan.hasNext()) {
i = scan.nextInt();
}
20190520
Java类集
1 List接口 内容允许重复 index+单值
使用List接口,则要通过其子类实例化对象,常用子类:ArrayList, Vector, LinkedList
1.1 ArrayList
List<String> a = new ArrayList<String>();
a.add("hello");
a.add(0, "tjut");
a.add(" world");
System.out.println(a); //[tjut, hello, world]
a.remove("world");
System.out.println(a); //[tjut, hello]
a.remove(0);
System.out.println(a); //[hello]
list.get(i);
list.size()
转化为array输出:
Object[] obj=list.toArray();
for(int i=0;i<list.size();i++) {
System.out.println(obj[i]);
}
String[] obj=list.toArray(new String[] {});
for(int i=0;i<list.size();i++) {
String s=obj[i];
System.out.println(s);
}
其它:
list.contains("hello");
list.subList(2, 4) 下表从0开始。包含2不包含4
1.2 Vector转化为List
List<String> list=new Vector<String>();
其它用法与ArrayList一致
1.3 ArrayList迭代器输出
Iterator it = list.iterator();//ListIterator it = list.ListIterator()
while(it.hasNext()) {//it.hasPrevious()
System.out.println(it.next());//it.previous(),反向输出
}
删除数据
while(it.hasNext()){
String s=it.next();
if("C".equals(s)){
it.remove();//list.remove(s); 错误
}
System.out.print(s+" ");
}
1.4 LinkedList链表
LinkedList<String> list=new LinkedList<String>();
list.add("A");
list.add("B");
list.add("C");
list.addFirst("X");
list.addLast("Y");
System.out.println(list);
System.out.println("链表头:"+list.getFirst());
System.out.println("链表尾:"+list.getLast());
list.poll()//弹出表头
2 Set接口 可接收多值的类,必须同时复写equals和hashCode.最好重写toString
常用的实现类HashSet, TreeSet。
2.1 HashSet 散列
package cn.tjut;
public class Person {
int age;
String name;
public Person(String name,int age) {
this.age = age;
this.name = name;
}
public boolean equals(Object s) {
if(s==this) {
return true;
}
if(!(s instanceof Person)) {
return false;
}
Person a = (Person)s;
if(a.age==this.age&&a.name.equals(this.name)) {
return true;
}
return false;
}
public int hashCode() {
return this.name.hashCode()*age;
}
@Override
public String toString() {
return name+age;
}
}
package cn.tjut;
import java.util.*;
class Demo{
public static void main(String[] args) {
Set<Person> a=new HashSet<Person>();
a.add(new Person("张三",30));
a.add(new Person("李二",20));
a.add(new Person("张三",30));
a.add(new Person("王五",50));
System.out.println(a); //[李二20, 王五50, 张三30]
}
}
2.2 TreeSet 需要实现Comparable接口
package cn.tjut;
import java.util.*;
class Demo{
public static void main(String[] args) {
Set<Person> a=new TreeSet<Person>();
a.add(new Person("张三",30));
a.add(new Person("李二",20));
a.add(new Person("张三",30));
a.add(new Person("王五",50));
System.out.println(a);
}
}
package cn.tjut;
public class Person implements Comparable{
int age;
String name;
public Person(String name,int age) {
this.age = age;
this.name = name;
}
public String toString() {
return name+age;
}
@Override
public int compareTo(Object o) {
Person b = (Person)o;
return this.age-b.age;
}
}
3 MAP接口
一对值
package cn.tjut.maspdemo;
import java.util.*;
public class Demo01 {
public static void main(String[] args) {
Map<String, Integer> map=new HashMap<String, Integer>();
map.put("zhangsan", 1);
map.put("zhangsan", 2);
map.put("lisi", 3);
map.put("wangwu", 5);
System.out.println(map);
Integer value=map.get("zhangsan");
System.out.println(value);
}
}
20190527
如果一个类能够排序,则一定要实现Comparable接口,也就是类的对象是可比较的。必须实现CompareTo()方法。
如果一个类能唯一确定元素,则以一定要实现HashCode方法equals()方法。
Properties类
Properties pro=new Properties();
pro.setProperty("BJ", "beijing");
pro.setProperty("TJ", "tianjin");
pro.setProperty("NJ", "nanjing");
System.out.println("属性存在:"+pro.getProperty("BJ"));
System.out.println("属性不存在:"+pro.getProperty("SH"));
System.out.println("属性不存在,指定默认值:"+pro.getProperty("SH","没有此属性。"));
把properties存到磁盘文件中
pro.store(new FileOutputStream(new File("E:"+File.separator+"demo.properties")), "Area Info");
pro.load(new FileInputStream(new File("E:"+File.separator+"demo.properties")));
Stack类 栈的特点是后进先出
Stack<String> s=new Stack<String>();
s.push("A");
s.push("B");
s.push("C");
s.push("D");
System.out.println(s.pop());
System.out.println(s.pop());
System.out.println(s.pop());
System.out.println(s.pop());
20190530,20190603
JDBC(import java.sql.*;)
package cn.tjut;
import java.sql.*;
class Demo{
public static final String DBDRIVER ="com.mysql.jdbc.Driver";
public static final String DBURL="jdbc:mysql://localhost:3306/test";
public static final String DBUSER = "root";
public static final String DBPASS = "";
public static void main(String[] args) throws Exception {
String sql1 = "insert into person values(1,'name3','woman',15)";
String sql2 = "insert into person values(?,?,?,?)";
Connection conn = DriverManager.getConnection(DBURL,DBUSER,DBPASS);
Statement stem = conn.prepareStatement();
stem.execute(sql1);//改动数据
stem.close();
conn.close();
String sql = "select * from person";
Connection conn = DriverManager.getConnection(DBURL,DBUSER,DBPASS);
Statement stem = conn.createStatement();
ResultSet res = stem.executeQuery(sql);
while(res.next()) {
int id = res.getInt("id");
String name = res.getString("name");
String sex = res.getString("sex");
int age = res.getInt("age");
System.out.println("ID:"+id+" NAME:"+name+" SEX:"+sex+" AGE:"+age);
}
stem.close();
conn.close();
PreparedStatement pstem = conn.prepareStatement(sql2);
pstem.setInt(1, 3);
pstem.setString(2, "name3");
pstem.setString(3, "woman");
pstem.setInt(4, 60);
pstem.executeUpdate();
pstem.close();
conn.close();
}
}
20190606
反射(import java.lang.reflect.*;)
package cn.tjut;
public class Person extends Sp implements Info,Contact{
private String name;
private int age;
public Person(){}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public Person(String name, int age) {
super();
this.name = name;
this.age = age;
}
public String toString() {
return "hello";
}
}
import java.lang.reflect.*;
class Demo{
public static void main(String[] args) throws Exception {
Class<?> c=null;
c=Class.forName("cn.tjut.Person");
Class<?>[] inter = c.getInterfaces();//获取接口
for(i=0;i<inter.length;i++){
System.out.println(inter[i]);
}
Package pack=c.getPackage();//获取包
System.out.println(pack.getName());
Class<?> sp=c.getSuperclass();//获取父类
System.out.println(sp.getName());
Constructor<?>[] cons=c.getConstructors();//获取构造方法
for(int i=0;i<cons.length;i++){
System.out.println(cons[i].getName());
};
Method[] me=c.getMethods();//获取普通方法
for(int i=0;i<me.length;i++){
System.out.println(me[i].getName());
};
}
}
打赏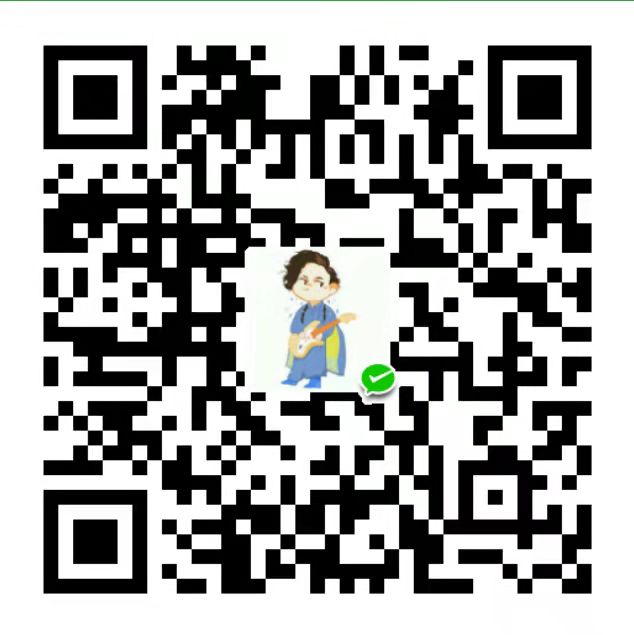

微信扫一扫,打赏作者吧~